Create a pull request conflict
Some merge conflicts may make it impossible for the source code to merge automatically. In that case, you’ll need to fix the issue manually.
To demonstrate how to merge with conflicts, we first need to create and edit code on multiple branches and attempt to merge them.
To start, we need to reproduce the situation where the conflict occurs. We can make a conflict happen using the equality operator ==
for one branch of sort.js and the strict equality operator ===
for the other.
Create a new repository and push the following source code to the main branch.
// sort.js
var number = [19, 3, 81, 1, 24, 21];
console.log(number);
Next, create two branches.
$ git checkout -b add-sort-func2
$ git checkout -b add-sort-func1
Then edit the source code on the add-sort-func1
branch.
// sort.js
var sortNumber = function (number) {
number.sort(function (a, b) {
if (a == b) {
return 0;
}
return a < b ? -1 : 1;
});
};
var number = [19, 3, 81, 1, 24, 21];
sortNumber(number);
console.log(number);
And commit and push the changes.
$ git add sort.js
$ git commit -m "<commit_message>"
$ git push origin add-sort-func1
“A process of sorting an array has been added”
Next, make a pull request for the add-sort-func1
branch and merge.
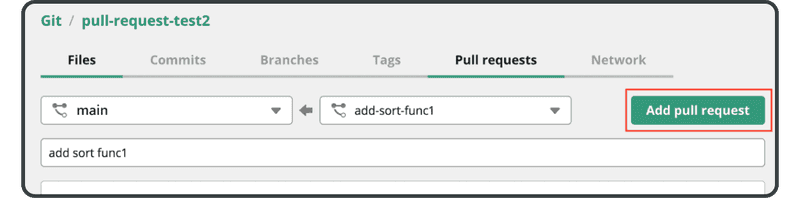
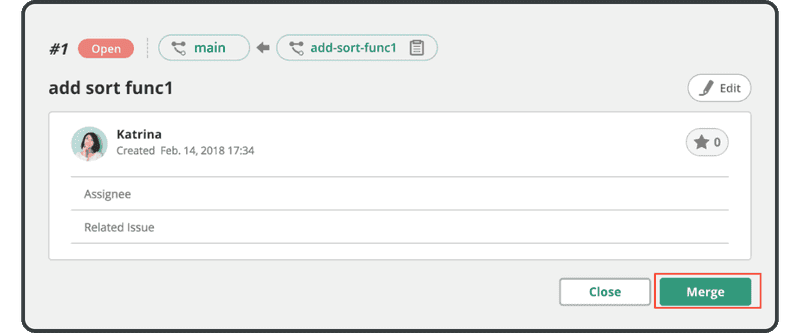
Then switch the branch to add-sort-func2
.
$ git checkout add-sort-func2
Edit the source code.
// sort.js
var sortNumber = function (number) {
number.sort(function (a, b) {
if (a === b) {
return 0;
}
return a < b ? -1 : 1;
});
};
var number = [19, 3, 81, 1, 24, 21];
sortNumber(number);
console.log(number);
And commit and push the changes.
$ git add sort.js
$ git commit -m "<commit_message>"
$ git push origin add-sort-func2
“A process of sorting an array has been added”
Next, create a pull request for the add-sort-func2
branch.
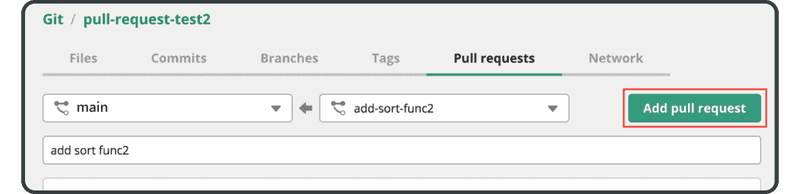
This is where our conflict occurs.
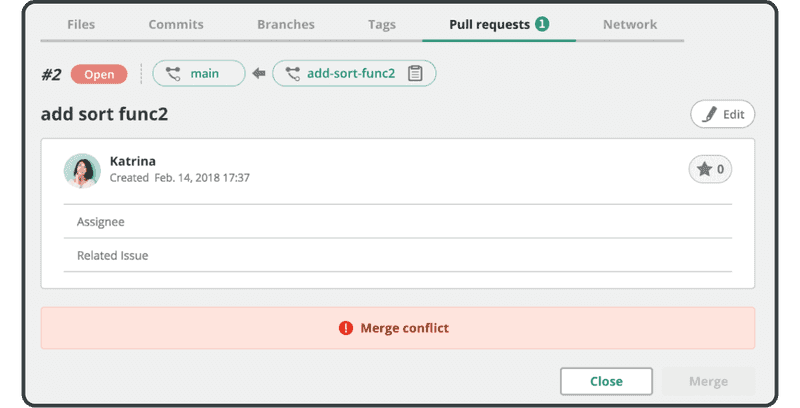
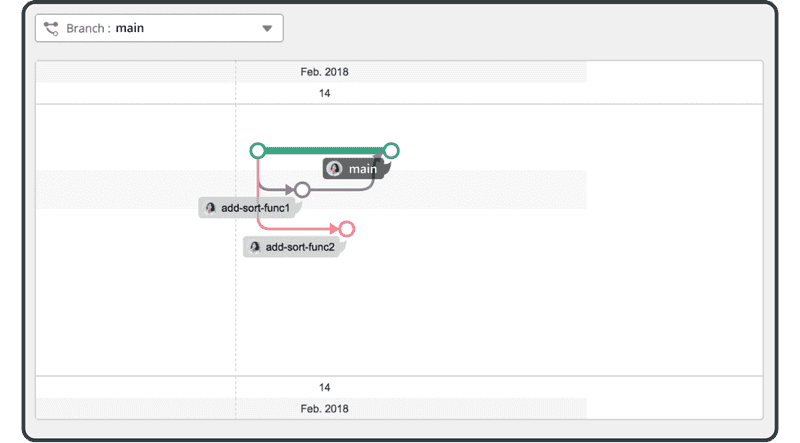